Introduction
The ISARA Radiate OpenSSL Connector lets you create Quantum Safe X.509 Multiple Public Key Algorithm Certificates and PKCS #10 Multiple Public Key Algorithm Certificate Signing Requests using the authentication algorithms provided in the ISARA Radiate Security Solution Suite.
For more information about ISARA quantum safe solutions, please visit https://www.isara.com.
This Tutorial assumes that you are already familiar with X.509 certificates, certificate signing requests, OpenSSL, and have extensive experience with them.
One of the design goals of our multiple public key algorithm certificate scheme was to make sure that integration into pre-existing systems would be simple. This can be seen in the new utilities and APIs that we added.
We created minimalist utilities that transform already existing certificate signing requests and X.509 certificates into their multiple public key algorithm variants. This means that if you already have scripts and infrastructure in place, you shouldn’t need to change any of your pre-existing commands; only add new ones. This also means that if you have created applications or libraries that use OpenSSL, you can begin integration by only inserting code and not changing your pre-existing code. This will be inefficient, but eases your prototyping. As efficiency becomes a priority, you can begin intermingling the code and removing redundancies.
The architecture for our multiple public key algorithm certificates is limited to creating, inserting and removing attributes and extensions into/from certificate signing requests and X.509 certificates. Since OpenSSL already provides APIs for doing these things to attributes and extensions, the only new additions to the OpenSSL API that are related to multiple public key algorithm certificates are for ASN.1 encoding and decoding of these new attributes and extensions. Details of how to use these new additions can be found in the Programmer’s Guide To Multiple Public Key Algorithm Certificates section below.
An important feature of MPKA certificates is that they are backwards compatible with systems that are not aware of MPKAC extensions. In situations where the system does not understand MPKAC extensions, those extensions will be ignored and the certificate will be processed the same as a traditional X.509 certificate.
The QS Multiple Public Key Algorithm Certificate Tutorial covers the following topics:
Introduction to the Authentication Schemes
The ISARA Radiate OpenSSL Connector adds the following quantum safe authentication schemes to OpenSSL:
-
Leighton-Micali Signature Scheme (LMS)
-
Dilithium Lattice-Based Signature Scheme
-
Rainbow Multivariate Signature Scheme
Note
|
The abbreviation in parentheses above is not an official algorithm abbreviation. It is the compact name of the algorithm used in the code of the ISARA Radiate OpenSSL Connector. |
Many of the schemes' details are abstracted via the EVP PKEY API. For details, please see ISARA Radiate OpenSSL Connector 1.3 Developer’s Guide.
Caveats
-
LMS keys can only produce a specific number of signatures. The total number of signatures available to an LMS private key depends on parameter selection.
-
LMS private keys require special care during the signing operation. A new pkey option called
lms_index
must be set to a unique OTS index. This is discussed below, but for more details, please see the User’s Guide. -
All our signature schemes only accept 512 bit (64 bytes) digests as input. This may be different from other implementations of these signature algorithms.
-
Our new X.509 multiple public key algorithm certificates will have 2 private keys associated with each certificate. This is not compatible with the
PKCS12_parse()
API which can only return a single private key per certificate.
Introduction to Generic X.509 Certificates and Certificate Signing Requests
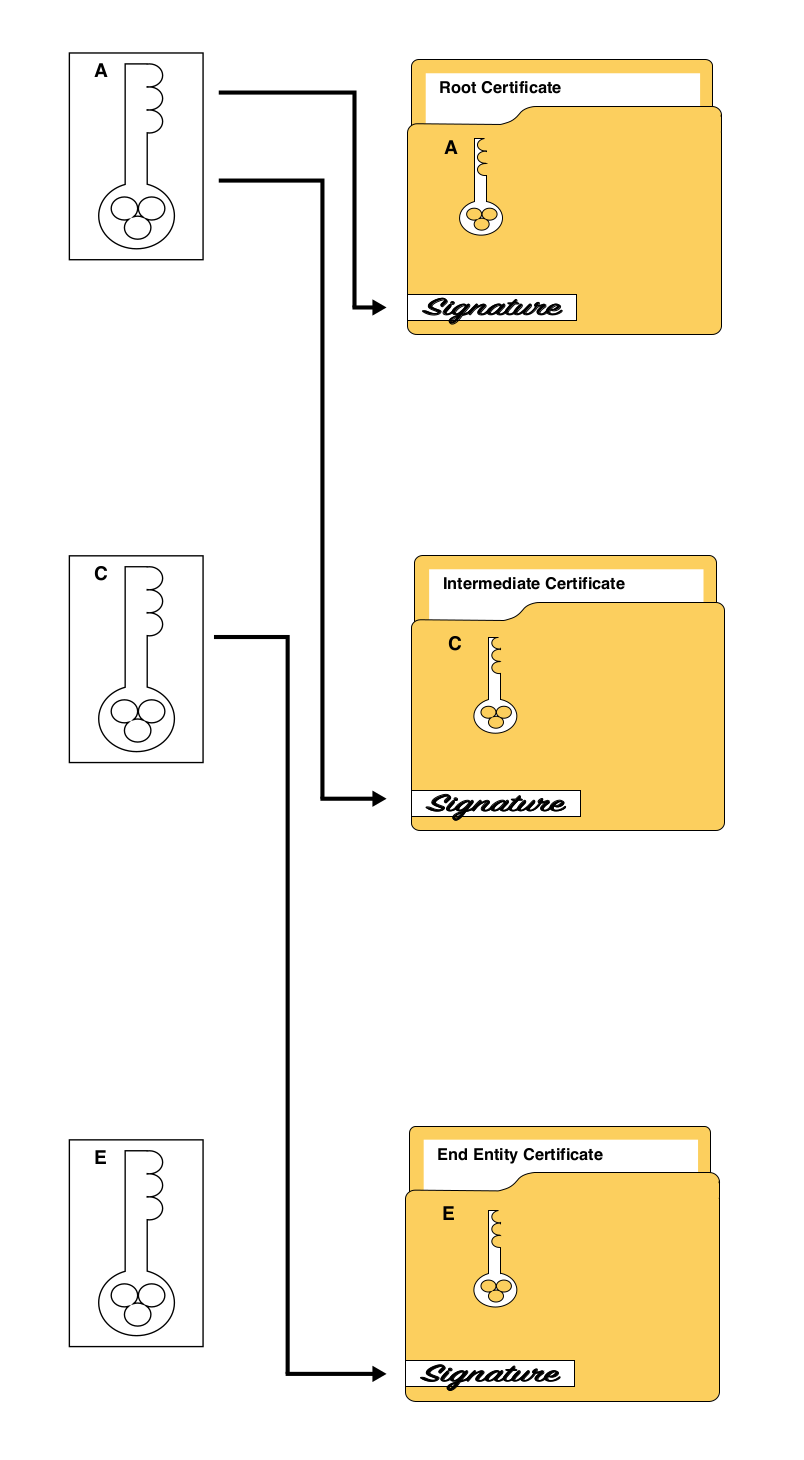
A detailed discussion of X.509 certificates, PKCS #10 certificate signing requests and their multiple public key algorithm variants is outside the scope of this document. More details about X.509 certifcates can be found in RFC-5280. More details about PKCS #10 certificate signing requests can be found in RFC-2986.
Generally speaking, each X.509 certificate must contain a subject identifier, a public key, a signature algorithm identifier, and a signature. The main purpose of the certificate is to bind the subject that is identified by the subject identifier to the public key that is in the certificate. This binding is certified by having a trusted third party sign the certificate with their private key and add that signature into the certificate. This third party then publishes their public key to let anyone verify the signature in the certificate. How does the third party gain their status as trusted? The simplest way is to publish their public key in a certificate that was signed by another "actually trusted" third party. How a third party gains the status of "actually trusted" is beyond the scope of this document.
The "actually trusted" third parties distribute their certificates as self-signed certificates. That is, the private key that signs the certificate is the one that corresponds to the public key in the certificate. These are known as root certificates.
Thus you could have a chain of certificates where each certificate is signed by another subject, called the issuer, and verifiable by the public key in the issuer’s certificate. If the top of this chain is a root certificate who’s subject was "actually trusted" then you would say that all certificates in that chain are trusted subject to other conditions of validity.
An issuer must bind the subject identified by the subject identifier to the public key. This is done by having the subject create a certificate signing request. Generally speaking, each certificate signing request must contain a subject identifier, a public key, a signature algorithm identifier and signature where the signature was created by the public key’s corresponding private key. This then proves possession of the private key. The subject sends the issuer the certificate signing request and the issuer verifies the signature. The issuer can then create a certificate containing the subject identifier and public key from the certificate signing request and sign that certificate with their private key and attach that signature to the newly created certificate.
Introduction to QS X.509 Multiple Public Key Algorithm Certificates and Certificate Signing Requests
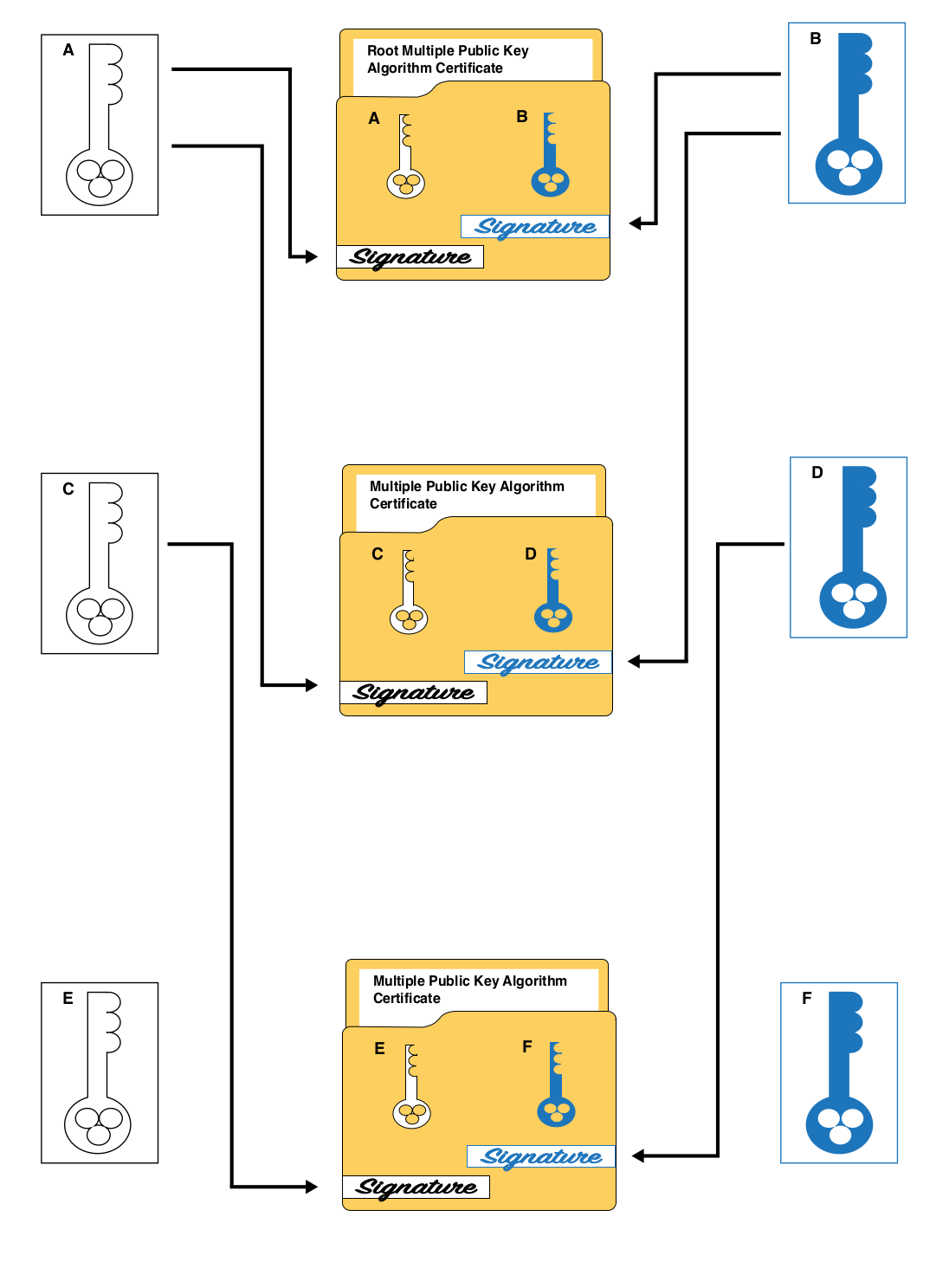
Each X.509 certificate contains a public key, a signature algorithm identifier, and a signature. As specified in RFC-5280, X.509 certificates may contain extensions. QS X.509 multiple public key algorithm certificates are simply X.509 certificates that contain newly defined extensions with a subject alternative public key, an alternative signature algorithm identifier, and an alternative signature. These all mirror the original formats and purposes from normal X.509 certificates. The only difference is that for our case, they will be associated with quantum safe authentication schemes.
Each certificate signing request contains a public key, a signature algorithm identifier, and a signature. As specified in RFC-2986, certificate signing requests may contain attributes. QS multiple public key algorithm certificate signing requests are simply certificate signing requests with newly defined attributes that contain a subject alternative public key, an alternative signature algorithm identifier, and an alternative signature. These all mirror the original formats and purposes from normal certificate signing requests. The only difference is that for our case, they will be associated with quantum safe authentication schemes.
Introduction to Our New Utilities
We provide a utility called reqQSExtend
that transforms a certificate
signing request into a multiple public key algorithm certificate.
We provide two different utilities to transform an X.509 certificate into a
multiple public key algorithm certificate. The first and simplest utility is
called x509QSDirectExtend
. This utility is meant for transforming self-signed
root certificates or quickly transforming experimental certificates directly
from public keys; no certificate signing request required. The second utility is
called x509QSExtend
and uses a multiple public key algorithm certificate
signing request to transform an X.509 certificate into its multiple public key
algorithm form.
We provide a utility called x509QSVerify
that does verification of the quantum
safe signatures in the certificates given a multiple public key algorithm
certificate chain.
For the utilities that accept quantum safe private keys as input to create
signatures, we always have the optional -lms_index index
parameter. If the
private key is not an LMS private key, the utility will return an error. This
parameter allows the user to set the index to an unused value ensuring that
the OTS is never used more than once.
For the utilities that accept private keys as input to create signatures, we
always have the optional -passin arg
or -passinqs arg
parameter. These
specify the key password source. For more information about the format of arg
please see the PASS PHRASE ARGUMENTS section in
openssl.
It is important to note that in order for the X.509 multiple public key algorithm certificates and multiple public key algorithm certificate signing requests to be quantum safe, the artifacts in the extensions and attributes must be associated with quantum safe signature schemes. This is enforced by these utilities.
reqQSExtend
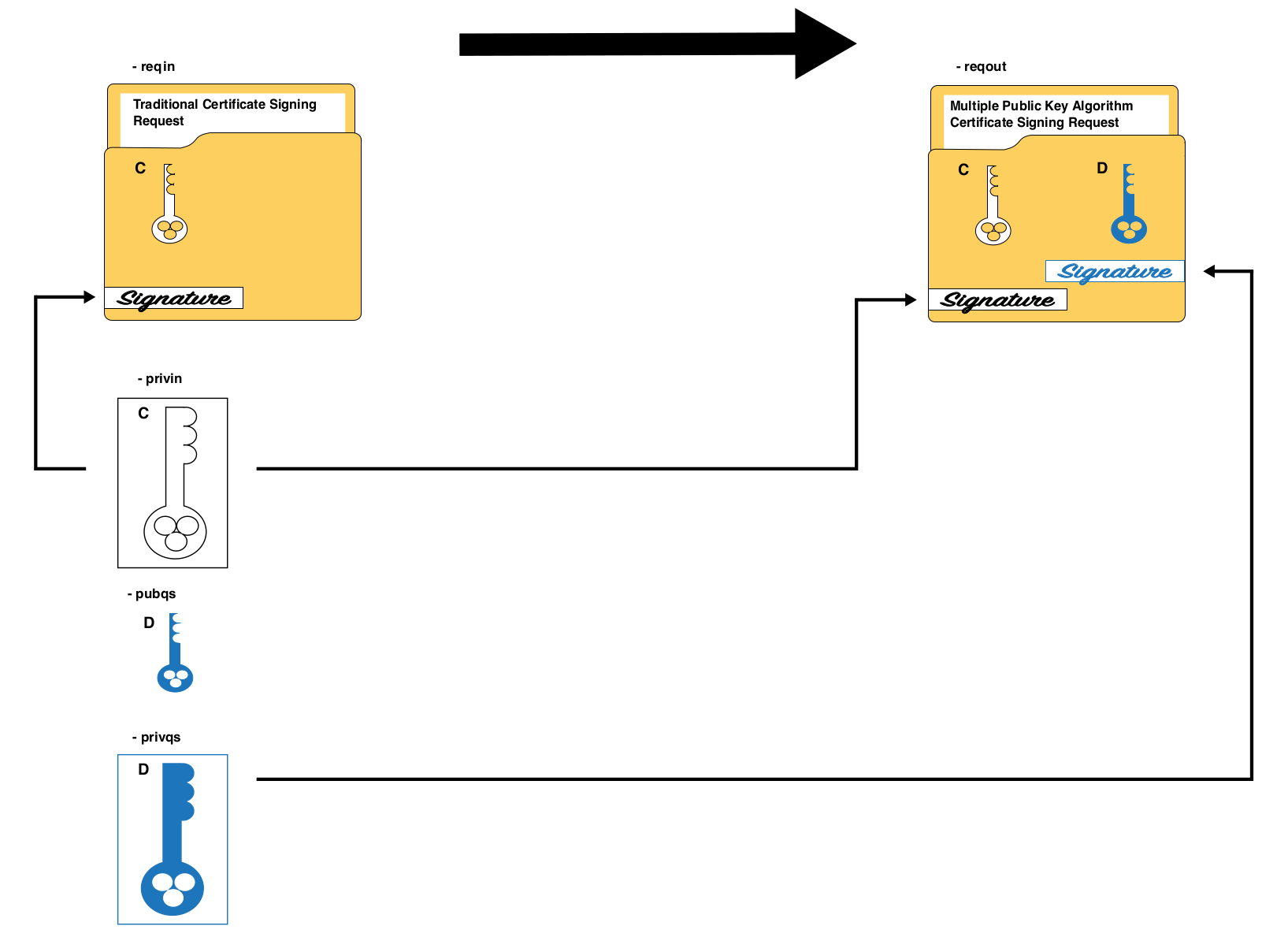
This utility transforms a previously generated certificate signing request into a quantum safe multiple public key algorithm certificate signing request by adding a subject alternative public key attribute, an alternative signature algorithm attribute, and an alternative signature attribute. The first attribute is created based on the quantum safe public key. This attribute is inserted into the certificate signing request. The alternative signature algorithm attribute is then created based on the private key and added to the certificate signing request. The alternative signature attribute is then generated by signing the certificate signing request and adding it to the certificate signing request. Finally, the certificate signing request is signed with the original private key.
Usage: openssl reqQSExtend [options]
where options may be
-engine e Use IQR Engine library <e>.
-reqin file The CSR in pem format.
-reqout file The CSR in pem format with new ALT extensions.
-privin file The private key used to sign the original CSR in pem format.
-pubqs file The public QS key.
-privqs file The private QS key.
-lms_index index In the case of LMS, the private key q index value.
-passin The private key password source.
-passinqs The private QS key password source.
x509QSDirectExtend
This utility transforms a previously generated X.509 certificate into a quantum safe X.509 multiple public key algorithm certificate by adding a subject alternative public key extention, an alternative signature algorithm extension and an alternative signature extension. The first extension is created based on the quantum safe public key. The second extension is created based on the quantum safe private key. These two extensions are then inserted into the X.509 certificate. Then the quantum safe signature is generated and transformed into an alternative signature extension and then added to the certificate. Finally, the X.509 certificate is signed with the original private key.
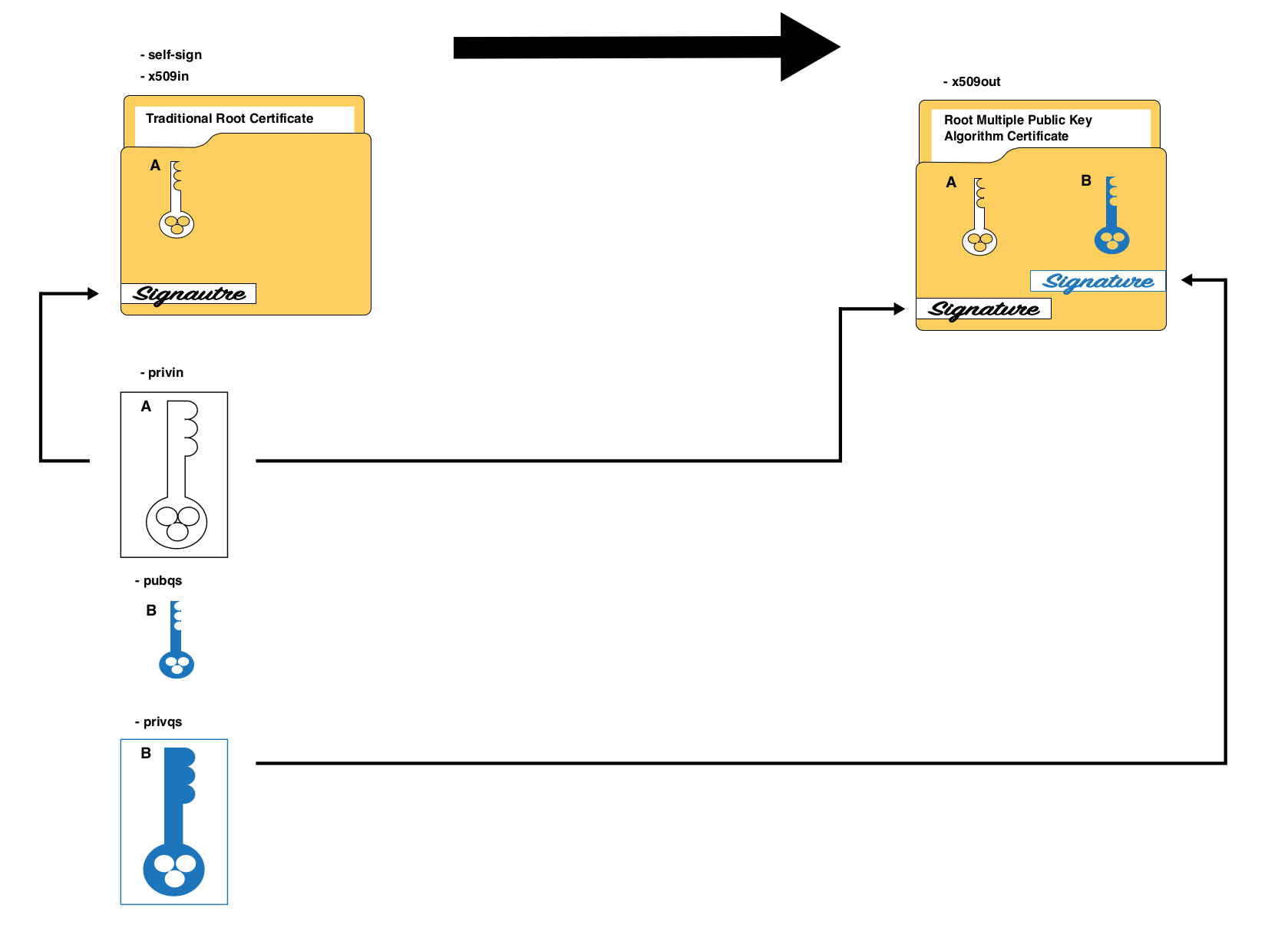
If you are using this utility because you want to create a self-signed root
certificate, you may not want to bother producing a separate public key file.
In that case, you can use the -self_sign
flag to specify that the public key
should be found in the file specified by -privqs
. If you use the -self_sign
flag you must not use the -pubqs
flag.
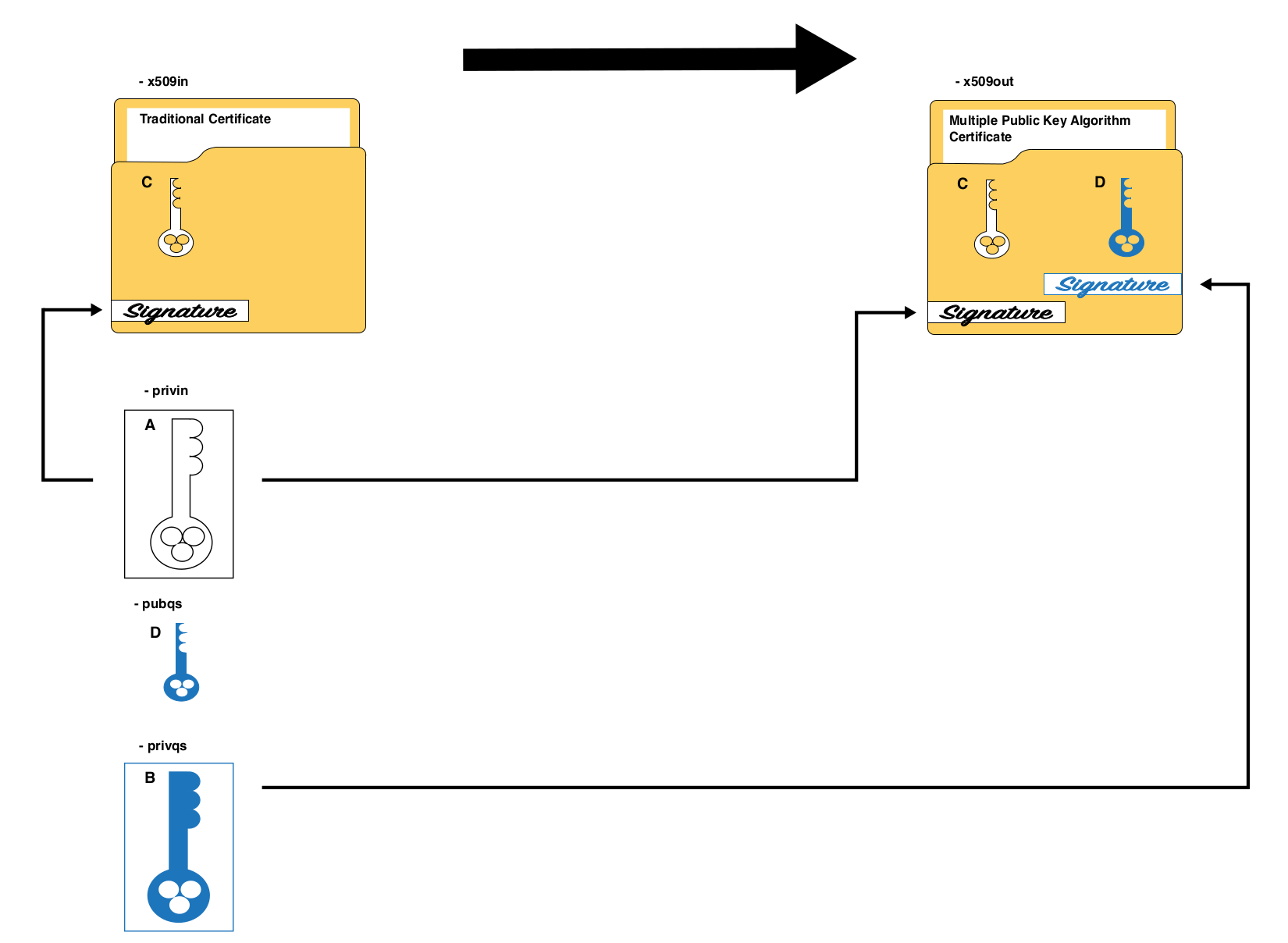
If you are using this utility because you want to quickly create an experimental
X.509 certificate skipping the step of creating a certificate signing request,
then you must not specify the -self_sign
flag and must use the -pubqs
flag
to specify a public key file.
Usage: openssl x509QSDirectExtend [options]
where options may be
-engine e Use IQR Engine library <e>.
-x509in file The X509 certificate in pem format.
-x509out file The X509 MPKA certificate in pem format with new ALT extensions.
-privin file The private key used to sign the original x509 certificate in pem format.
-pubqs file The public QS key. Incompatible with -self_sign.
-privqs file The private QS key.
-lms_index index In the case of LMS, the private key q index value.
-self_sign The public key should be obtained from the private key. Incompatible with -pubqs.
-passin The private key password source.
-passinqs The private QS key password source.
x509QSExtend
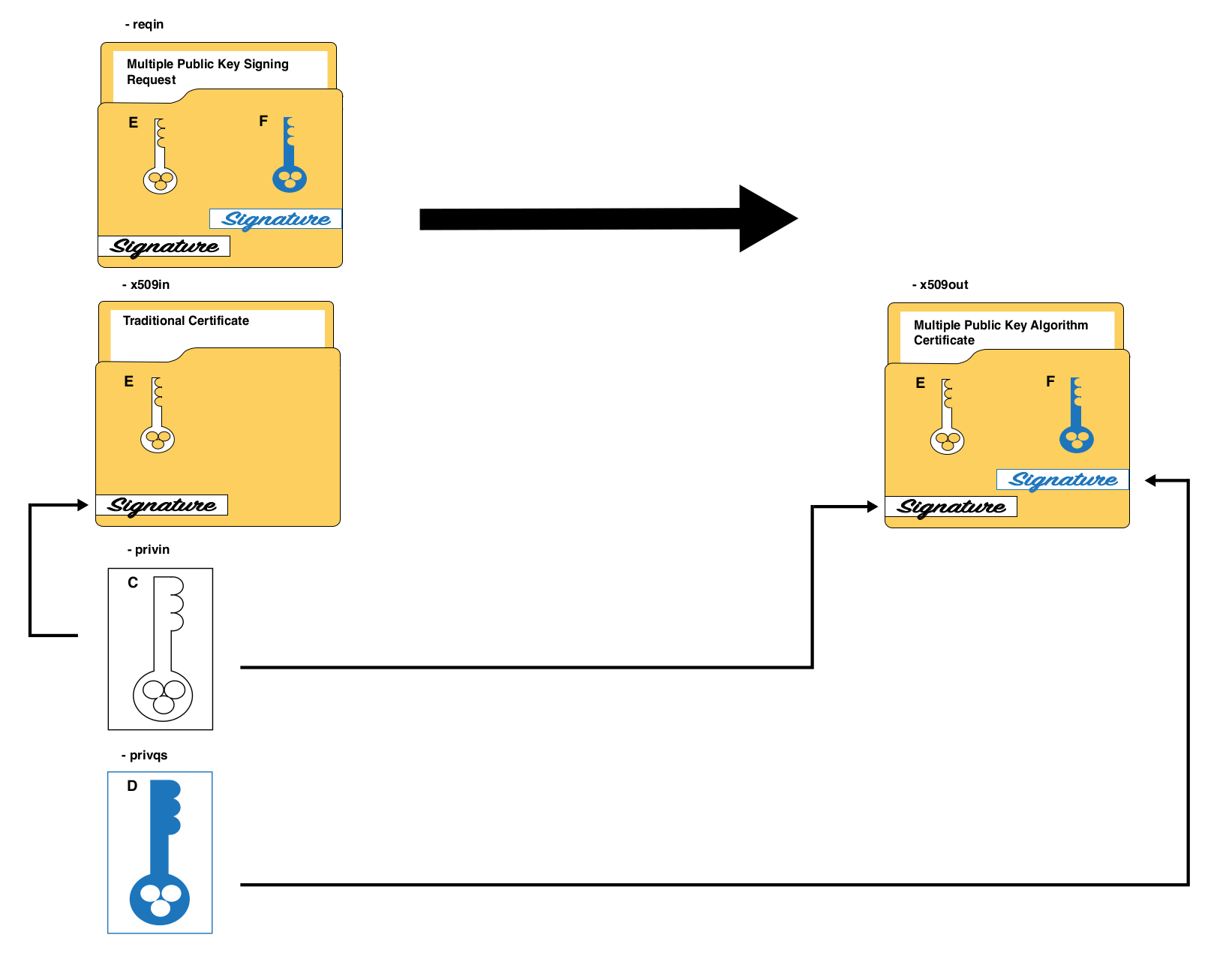
This utility transforms a previously generated X.509 certificate into a quantum safe X.509 multiple public key algorithm certificate by adding a subject alternative public key extention and an alternative signature algorithm extension and then generating an alternative signature extension. The first two extensions are obtained from the quantum safe certificate signing request. The subject alternative public key attribute and alternative signature identifier attribute are transformed into X.509 extensions and inserted into the X.509 certificate. Then the quantum safe signature is generated using the quantum safe private key and transformed into an alternative signature extension and then added to the certificate. Finally, the X.509 certificate is signed with the original private key.
Usage: openssl x509QSExtend [options]
where options may be
-engine e Use IQR Engine library <e>.
-x509in file The X509 certificate in pem format.
-x509out file The X509 MPKA certificate in pem format with new ALT extensions.
-privin file The private key used to sign the original x509 certificate in pem format.
-reqin file The certificate signing request containing the ALT public key extension.
-privqs file The private QS key.
-lms_index index In the case of LMS, the private key q index value.
-passin The private key password source.
-passinqs The private QS key password source.
x509QSVerify
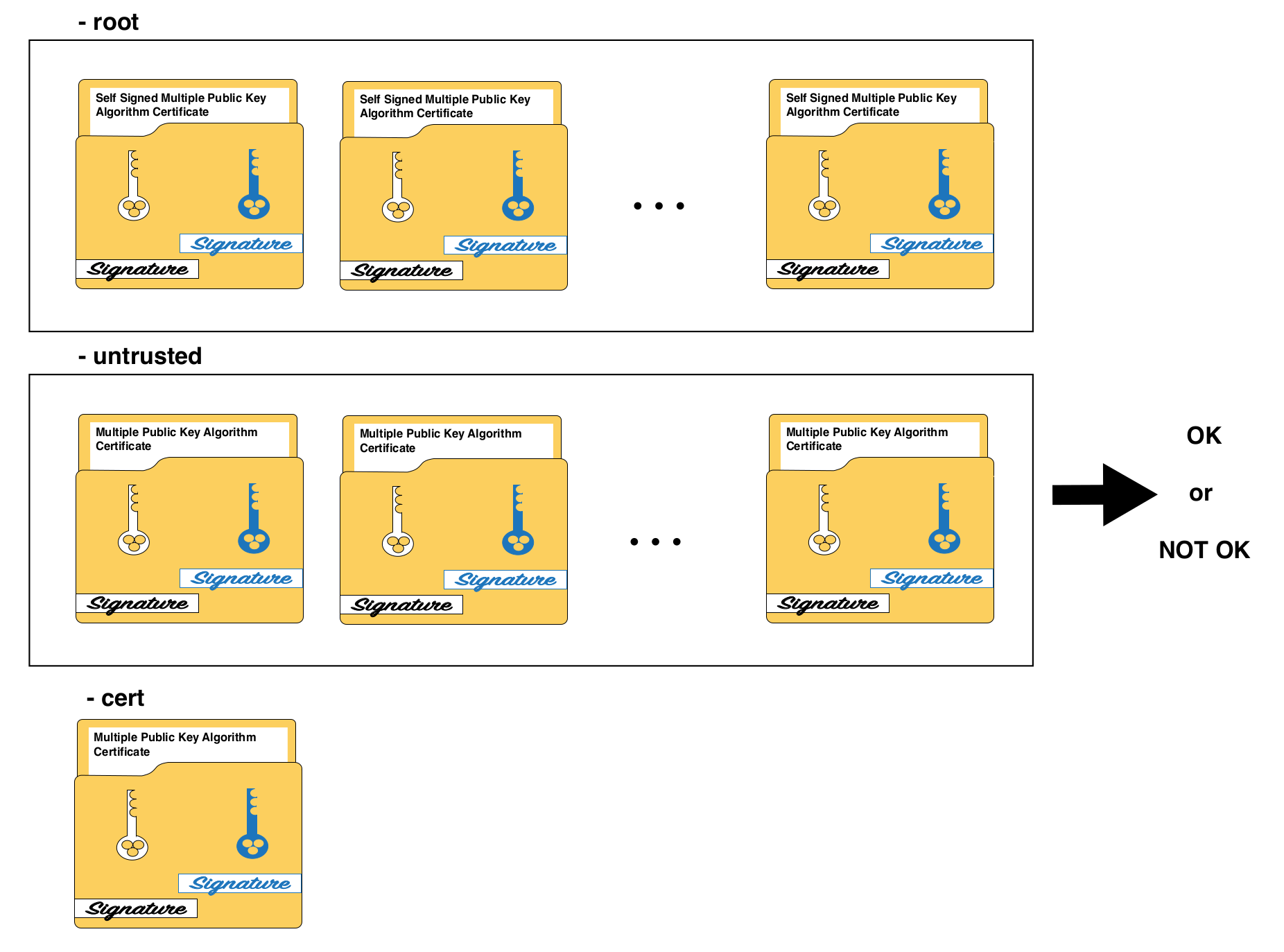
This utility primarily shows how to perform quantum safe signature verification
of X.509 multiple public key algorithm certificate chains. It is important to
note that this utility does not do verification of the normal signatures in
the X.509 certificates in the chain and only checks the validity of the
quantum safe signatures in the chain. However, the vanilla openssl verify
utility can still function correctly with quantum safe X.509 multiple public key
algorithm certificates as the new extensions are optional and will be ignored by
openssl verify
. In short, this utility was meant to complement
openssl verify
, not replace it.
Usage: openssl x509QSVerify [options]
where options may be
-engine e Use IQR Engine library <e>
-root file The self signed X509 root certificates concatenated into a single file.
-untrusted file All the untrusted certificates concatenated into a single file.
-cert file The certificate to be verified.
Programmer’s Guide To Multiple Public Key Algorithm Certificates
What follows is a list of operations you might want to do in your applications related to transforming certificates and certificate signing requests into their multiple public key algorithm variants.
Each section will begin with a description of what can be accomplished and where you can find the full source code to get more details.
Adding MPKA Attributes to CSRs
To learn how to create and add a subject alternative public key information
attribute, an alternative signature algorithm identifier attribute and an
alternative signature attribute to a certificate signing request please see
create_SAPKI_ATTRIBUTE()
, create_ALTSIGALG_ATTRIBUTE()
and
create_ALTSIG_ATTRIBUTE()
in reqQSExtend.c
.
Parsing MPKA Attributes in CSRs
To learn how to parse an X509_ATTRIBUTE
to get a SUBJECT_ALT_PUBLIC_KEY_INFO
please see the get_SAPKI_from_ATTRIBUTE()
function in x509QSExtend.c
.
To learn how to use the get_SAPKI_from_ATTRIBUTE()
function to obtain an
EVP_PKEY
from a multiple public key algorithm certificate signing request
please find where it is called in x509QSExtend.c
.
To learn how to parse an X509_ATTRIBUTE
to get an ASN1_BIT_STRING
which is
the actual alternative signature please see the get_ALTSIG_from_ATTRIBUTE()
function in x509QSExtend.c
.
To learn how to parse an X509_ATTRIBUTE
to get an X509_ALGOR
which is the
alternative signature algorithm identifier please see the
get_ALTSIGALG_from_ATTRIBUTE()
function in x509QSExtend.c
.
MPKA Verification of a CSR
To learn how to use the functions above and the EVP_PKEY
that
is the subject alternative public key to verify the certificate signing request
using the quantum safe signature scheme, please find where they are called in
x509QSExtend.c
.
Adding MPKA Extensions to X.509 Certificates
You can create the various alternative extensions using the X509V3_EXT_i2d()
function. Please see how it is used in x509QSExtend.c
.
Parsing MPKA Extensions in X.509 Certificates
To learn how to get the subject alternative public key as an EVP_PKEY
from an
X.509 multiple public key algorithm certificate, please see
get_SAPKI_pubkey()
in x509QSVerify.c
.
MPKA Verification of an X.509 Certificate
To learn how to write a callback function that verifies a signature of a
certificate using a quantum safe signature scheme, please see the
qs_verification_cb()
function in x509QSVerify.c
. This callback would be
passed into OpenSSL via X509_STORE_set_verify_cb()
.
TLS and MPKA X.509 Certificates
New APIs are also added to OpenSSL’s libssl library in order to facilitate the usage of multiple public key algorithm certificates for TLS secure handshake.
To load multiple public key algorithm certificates into the SSL context for peer authentication during TLS secure handshake, no change is needed regarding libssl API usage (i.e., existing APIs can continue to be used).
For instance, to load the end entity certificate and any accompanying intermediate CA certificates needed to form the complete certificate chain into the SSL context, for the purpose of sending them to the peer for entity authentication, the following function can be used.
int SSL_CTX_use_certificate_chain_file(SSL_CTX *ctx, const char *file);
It loads a multiple public key algorithm certificate chain from file
into
ctx
. The certificates must be in PEM format and must be sorted starting with
the end entity certificate (actual client or server certificate), followed by
intermediate CA certificates, if any. For more details on this function, and
other similar functions, please refer to OpenSSL manpages
(https://www.openssl.org/docs/man1.0.2/).
To load root CA certificates into the SSL context, for the purpose of verifying peer’s certificates, the following function can be used.
int SSL_CTX_load_verify_locations(SSL_CTX *ctx, const char *CAfile, const char *CApath);
It loads multiple public key algorithm certificates specified by CAfile
and/or CApath
as trusted CA certificates for ctx
. For more details on this
function, please refer to OpenSSL manpages
(https://www.openssl.org/docs/man1.0.2/).
To load private keys into the SSL context for operations such as digital
signature creation during TLS secure handshake, new APIs are added to libssl
for loading QS private keys.
Each multiple public key algorithm certificate corresponds to two private keys, one (the classical private key) corresponding to the subject public key in the subject public key info of the certificate, and the other (the QS private key) corresponding to the subject alternative public key in the extension of the certificate.
The classical private key can be loaded into the SSL context using existing
libssl functions such as SSL_CTX_use_PrivateKey()
. To load the QS private
key, the following new functions are introduced.
int SSL_CTX_use_ALTPrivateKey(SSL_CTX *ctx, EVP_PKEY *pkey);
This function loads pkey
as a private key to ctx
. Note that the private
keys must correspond to the end entity certificate loaded into the SSL context.
int SSL_CTX_use_ALTPrivateKey_ASN1(int type, SSL_CTX *ctx, const unsigned char *d, long len);
This function loads the private key of type type
stored at memory location
d
with length len
to ctx
. The private key types for QS private keys for
end entity certificate can be EVP_PKEY_DILITHIUM
for the Dilithium
lattice-based signature scheme or EVP_PKEY_RAINBOW
for the Rainbow
multivariate signature scheme. For a complete list of private key types, please
see OpenSSL header file evp.h
.
Note that Leighton-Micali signature scheme (LMS) can only be used for CA
certificates, and not for end entity certificates (i.e., LMS cannot be used for
SubjectAltPublicKeyInfo
for an end entity certificate). Therefore, it is
incorrect to load LMS private keys which have type EVP_PKEY_LMS
.
int SSL_CTX_use_ALTPrivateKey_file(SSL_CTX *ctx, const char *file, int type);
This function loads the first private key found in file
to ctx
.
The formatting type type
must be either SSL_FILETYPE_PEM
or
SSL_FILETYPE_ASN1
.
All of the three new functions return 1 on success. Otherwise, check the error stack to find out the error reason.
For an example of how these APIs are used in establishing a secure TLS
connection, please see our demo code openssl_tls.h
, openssl_server.c
, and
openssl_client.c
.
Legal
The ISARA Radiate OpenSSL Connector Binaries are licensed for use:
Copyright © 2017, ISARA Corporation, All Rights Reserved.
The code and other content set out herein is not in the public domain, is considered a trade secret and is confidential to ISARA Corporation. Use, reproduction or distribution, in whole or in part, of such code or other content is strictly prohibited except by express written permission of ISARA Corporation. Please contact ISARA Corporation at info@isara.com for more information.